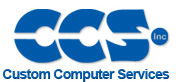 |
 |
View previous topic :: View next topic |
Author |
Message |
Kimi
Joined: 30 Jan 2005 Posts: 23 Location: Argentina
|
Search bytes in array |
Posted: Wed Feb 02, 2005 9:54 am |
|
|
Hello...
I need to search for a specific byte into a array [an array of 70 elements] . In fact, I have to detect a ',' and then look for the next one. Then copy the string into "comas" into a another string...
How can I do this efficiently? Now I'm using a if else structure, which is slow, and large...
Thanks
Kimi |
|
 |
valemike Guest
|
use a state machine |
Posted: Wed Feb 02, 2005 10:19 am |
|
|
It's pretty much a state machine that you initialize once. Index thru the array from the beginning; Once you find the semicolon, then you have to
go to state STATE_WAIT_FOR_DESIRED_LETTER. Once you find the desired letter, do what you have to do.
static int initialized = 0;
static int index;
if (!initialized)
{
state = STATE_WAIT_FOR_SEMICOLON;
index = 0;
}
switch (state)
{
case STATE_WAIT_FOR_SEMICOLON:
...
// index thru the array looking for a semicolon
break;
case STATE_WAIT_FOR_DESIRED_LETTER:
// index thru the remainder of the array looking for the desired character
break;
default:
break;
} |
|
 |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Wed Feb 02, 2005 10:36 am |
|
|
It sounds like you're either parsing a NMEA GPS message or
possibly you're doing something with a CSV format file (but
that would likely be done on a PC).
If it's the NMEA message, then here's some old code I wrote
many years ago. It might give you some ideas, or some
ideas of what not to do.
http://www.ccsinfo.com/forum/viewtopic.php?t=4402
Also, you can go to Google and search for this string:
NMEA int char
This will give you various sample code for parsing NMEA messages. |
|
 |
rwyoung
Joined: 12 Nov 2003 Posts: 563 Location: Lawrence, KS USA
|
|
Posted: Wed Feb 02, 2005 10:37 am |
|
|
Have you looked at strstr()? Would that work for you? _________________ Rob Young
The Screw-Up Fairy may just visit you but he has crashed on my couch for the last month! |
|
 |
Mark
Joined: 07 Sep 2003 Posts: 2838 Location: Atlanta, GA
|
|
Posted: Wed Feb 02, 2005 12:26 pm |
|
|
Here is some code you can look at. Originally this function would accept a string of break characters. In the second function, I modified it to accept only a single character. The functions also test for valid pointers. You could save even more space by removing these tests.
Code: |
#include <16F876.h>
#use delay(clock=4000000)
#fuses NOWDT, PUT
#define NULL 0
char test[]={"this,is,a,token,test"};
/* *************************************************************************
DESCRIPTION: You pass this function a string to parse, a buffer to
receive the "token" that gets scanned, the length of the
buffer, and a string of "break" characters that stop the
scan. It will copy the string into the buffer up to any of
the break characters, or until the buffer is full, and will
always leave the buffer null-terminated. It will return a
pointer to the first non-breaking character after the one
that stopped the scan.
RETURN: It will return a pointer to the first non-breaking character
after the one that stopped the scan or NULL on error or end of
string.
ALGORITHM: none
*************************************************************************** */
char *stptok(
char *s, /* string to parse */
char *tok, /* buffer that receives the "token" that gets scanned */
int8 toklen, /* length of the buffer */
char *brk) /* string of break characters that will stop the scan */
{
char *lim; /* limit of token */
char *b; /* current break character */
/* check for invalid pointers */
if (!s || !tok || !brk)
return (NULL);
lim = tok + toklen - 1;
while (*s && tok < lim)
{
for (b = brk; *b; b++)
{
if ((*s == *b) || (*s == '\r') || (*s == '\n'))
{
*tok = 0;
for (++s, b = brk; *s && *b; ++b)
{
if (*s == *b)
{
++s;
b = brk;
}
}
if (!*s)
return (NULL);
return (char *)s;
}
}
*tok++ = *s++;
}
*tok = 0;
if (!*s)
return (NULL);
return (char *)s;
}
/* *************************************************************************
DESCRIPTION: You pass this function a string to parse, a buffer to
receive the "token" that gets scanned, the length of the
buffer, and a "break" character that will stop the
scan. It will copy the string into the buffer up to any of
the break characters, or until the buffer is full, and will
always leave the buffer null-terminated. It will return a
pointer to the first non-breaking character after the one
that stopped the scan.
RETURN: It will return a pointer to the first non-breaking character
after the one that stopped the scan or NULL on error or end of
string.
ALGORITHM: none
*************************************************************************** */
char *stptok2(
char *s, /* string to parse */
char *tok, /* buffer that receives the "token" that gets scanned */
int8 toklen, /* length of the buffer */
char brk) /* break character that will stop the scan */
{
char *lim; /* limit of token */
/* check for invalid pointers */
if (!s || !tok)
return (NULL);
lim = tok + toklen - 1;
while (*s && tok < lim)
{
if ((*s == brk) || (*s == '\r') || (*s == '\n'))
{
*tok = 0;
s++;
if (*s)
{
if (*s == brk)
s++;
}
if (!*s)
return (NULL);
return (char *)s;
}
*tok++ = *s++;
}
*tok = 0;
if (!*s)
return (NULL);
return (char *)s;
}
void main (void)
{
char buf[10];
char *ptr_next;
char brk[]={","};
/* Initialize our pointer to our test string */
ptr_next = test;
/* Each time we call the function it will return a pointer to the rest of
the string. At some point, it will return NULL indicating that we are
done */
while(ptr_next)
{
ptr_next = stptok(ptr_next,buf,10,brk);
/* buf now contains the first word. Each time through the loop, buf will
be loaded with the next word */
}
/* Here I optimized a bit so that only 1 char can be passed in as
a break character */
ptr_next = test;
while(ptr_next)
{
ptr_next = stptok2(ptr_next,buf,10,',');
}
while(1 ) ;
}
|
|
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|