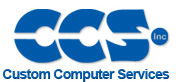 |
 |
View previous topic :: View next topic |
Author |
Message |
PCM programmer
Joined: 06 Sep 2003 Posts: 21708
|
|
Posted: Wed Aug 04, 2010 2:13 pm |
|
|
I heavily modified the program and was able to make it work.
1. I changed the program so it would work with the switches, etc., on
my PicDem2-Plus board. This involved changing the pushbutton pin to
Pin B0, and changing the LED indicator to a sending a char out the serial
port, etc.
2. Apparently on this PIC, you don't read the Port to clear the "mismatch
condition". You have to clear the corresponding bit in the IOCBF register.
I added a macro to do that, called clear_IOCBF0(). It clears bit 0 of the
IOCBF register.
3. I got rid of most of your code in the #int_rb isr because I just wanted
to get this thing working. It does work now, and when I press the
button on pin B0, I get the following output on the terminal window.
The count increments once per button press.
4. I changed the interrupt style so it would only interrupt on one edge
instead of both edges. That way, there is only one interrupt per button
press.
5. I had to add a delay of 10 ms in the isr, to suppress bounce in the
pushbutton. In my particular PicDem2-Plus board, I did previously
remove the capacitor on the Pin B0 push-button. It's possible that if
I put it back in, then the switch bounce problem might go away.
But you either need to debounce the switch in software with a delay, or
you need to debounce it in hardware. If you don't, you'll get multiple
interrupts per edge, sometimes.
Code: |
#include <16F1937.h>
#fuses INTRC_IO, NOWDT, PUT, BROWNOUT
#use delay(clock=4000000)
#use rs232(BAUD=9600, xmit=PIN_C6, rcv=PIN_C7, ERRORS)
#byte INTCON = 0x0B
#bit IOCIF = INTCON.0
#define clear_int_rb() IOCIF = 0
#byte IOCBF = 0x396
#bit IOCBF0 = IOCBF.0
#define clear_IOCBF0() IOCBF0 = 0
//--------------------------------------------
#int_rb
void RB_ISR(void)
{
static int8 count = '0';
putc(count);
count++;
delay_ms(10);
clear_IOCBF0();
}
//=======================================
void main(void)
{
int8 temp;
printf("Start \n\r");
port_b_pullups(0x01); // Enable pullup on pin B0.
delay_us(10); // Allow time for pullup to actually pull up
clear_IOCBF0(); //Clear any prexisting mismatch condition
clear_int_rb(); // Clear any pre-existing interrupt
enable_interrupts(INT_RB0_L2H); // Enable interrupt on release of pushbutton
enable_interrupts(GLOBAL);
while (1);
} |
|
|
 |
kjankows
Joined: 03 Aug 2010 Posts: 9
|
|
Posted: Wed Aug 04, 2010 2:29 pm |
|
|
PCM programmer,
Thank you very much. I understand what is going on. You have helped me to understand several things during this. Hopefully someone else will find it helpful as well.
Thanks again,
Kevin |
|
 |
jpapu
Joined: 21 Aug 2010 Posts: 3
|
|
Posted: Sat Aug 21, 2010 1:37 pm |
|
|
I am using the PIC16F727 and make use of the IOCB register. I use RB1 and RB2 to generate interrupts. First you have to make sure that you set BIT7 and BIT3 are set high in the INTCON register. Next you have to use have to create a interrupt service routine that will handle the change on PORTB RB1 or RB2. Use the CCS command #INT_RB at the beginning of the service routine. This is where the PIC will jump to when it is going to service a PORTB change interrupt. Once you jump to service the interrupt perform the code you need to service the interrupt. Before you leave the ISR make sure clear BIT 0 in INTCON. This is the PORTB change interrupt flag and it must be cleared in software.
Hope that helps. |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|